Ví dụ như bạn có 1 ký tự 52.2 m² và bạn muốn lấy 52.2 sau đó lưu vào database.
Certainly! Let’s break down the regular expression and the code:
The Regular Expression: (\d+(\.\d+)?)\s*m?
(\d+(\.\d+)?)
- This is the main capturing group that captures the area value.
\d+
: Matches one or more digits (0-9). This will match whole numbers.(\.\d+)?
: This is a nested capturing group that matches a decimal point followed by one or more digits. The?
at the end makes this whole group optional, so the regex can match both whole numbers and decimal numbers.\.
: Matches a literal decimal point.\d+
: Matches one or more digits after the decimal point.
\s*
- Matches zero or more whitespace characters. This allows for potential spaces between the number and the “m”.
m?
- Matches the letter ‘m’ zero or one time. The
?
makes it optional. This can be useful if sometimes the input might just say “52.2m” instead of “52.2 m²”.
The Code:
areaRegex := regexp.MustCompile(
(\d+(.\d+)?)\s*m?)
- This line compiles the regular expression.
MustCompile
will panic if the regex does not compile correctly, ensuring that invalid patterns are caught during program initialization.
areaMatch := areaRegex.FindStringSubmatch(str)
FindStringSubmatch
searches for the pattern in the provided string and returns the matches in a slice. The first item in the slice (areaMatch[0]
) is the entire matched string, and the subsequent items are the captured groups. In our case,areaMatch[1]
will contain the area value we’re interested in.
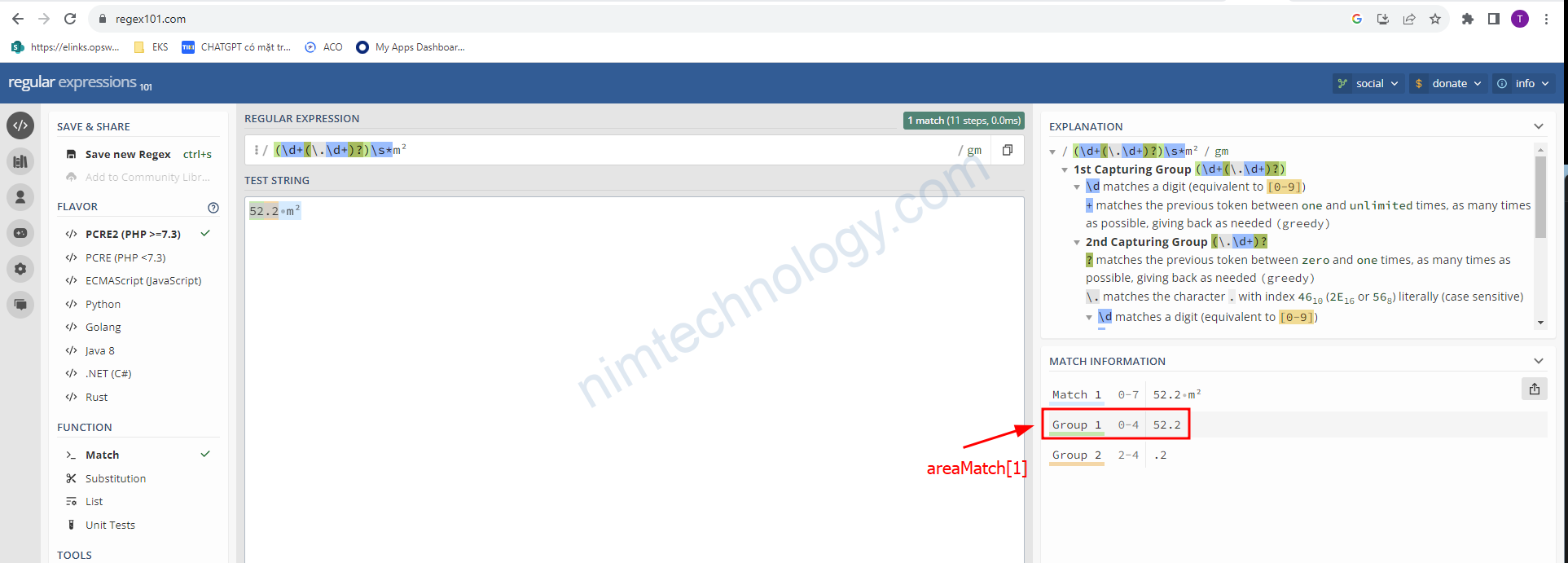
if len(areaMatch) > 0 { ... }
- Checks if there was a match. If there was a match, the length of
areaMatch
will be greater than 0.
fmt.Println("Area:", areaMatch[1])
- Prints the captured area value.
So, when the string “52.2 m²” is processed with this code, the regular expression will recognize “52.2” as the area value and print it out.