Nếu Sếp bảo bạn giờ anh muốn em code 1 script restart pod trên k8s khi nó bị freeze.
Còn làm sao biết là pod đó bị freeze thì call vào 1 enpoint.
Chà viêt Script hả ta. No No em code GO.
Bạn có thể tham khảo
Đây là code của anh trai:
package main import ( "context" "flag" "fmt" metav1 "k8s.io/apimachinery/pkg/apis/meta/v1" "k8s.io/client-go/kubernetes" "k8s.io/client-go/tools/clientcmd" ) func main() { kubeconfig := flag.String("kubeconfig", ".kube/config", "location to your kubeconfig file") config, err := clientcmd.BuildConfigFromFlags("", *kubeconfig) if err != nil { } clientset, err := kubernetes.NewForConfig(config) if err != nil { } ctx := context.Background() pods, err := clientset.CoreV1().Pods("default").List(ctx, metav1.ListOptions{}) if err != nil { } fmt.Println(pods) fmt.Println("Pods from default namespace") for _, pod := range pods.Items { fmt.Printf("%s", pod) } deployments, err := clientset.AppsV1().Deployments("default").List(ctx, metav1.ListOptions{}) fmt.Println("Deployments from default namespace") for _, deployment := range deployments.Items { fmt.Printf("%s", deployment) } }
Code của chatGPT
To use a service account, you need to first create a service account in your Kubernetes cluster, and then create a Kubernetes clientset using the service account token and CA certificate.
Here is an example Go code snippet that uses a service account to restart a pod with a given name and namespace:
package main import ( "context" "fmt" "os" "k8s.io/apimachinery/pkg/api/errors" metav1 "k8s.io/apimachinery/pkg/apis/meta/v1" "k8s.io/client-go/kubernetes" "k8s.io/client-go/rest" "k8s.io/client-go/tools/clientcmd" ) func main() { // Load in-cluster kubeconfig if running inside Kubernetes cluster, // otherwise use the kubeconfig file in the default location var config *rest.Config if _, err := os.Stat("/var/run/secrets/kubernetes.io/serviceaccount/token"); err == nil { config, err = rest.InClusterConfig() if err != nil { panic(err.Error()) } } else { kubeconfig := os.Getenv("HOME") + "/.kube/config" config, err = clientcmd.BuildConfigFromFlags("", kubeconfig) if err != nil { panic(err.Error()) } } // Create Kubernetes clientset using service account token and CA certificate clientset, err := kubernetes.NewForConfig(config) if err != nil { panic(err.Error()) } // Set pod name and namespace podName := "my-pod" podNamespace := "default" // Get pod with given name and namespace pod, err := clientset.CoreV1().Pods(podNamespace).Get(context.Background(), podName, metav1.GetOptions{}) if err != nil { if errors.IsNotFound(err) { fmt.Printf("Pod %s in namespace %s not found\n", podName, podNamespace) } else { panic(err.Error()) } } else { // Restart pod by deleting and creating a new pod with the same spec err = clientset.CoreV1().Pods(podNamespace).Delete(context.Background(), podName, metav1.DeleteOptions{}) if err != nil { panic(err.Error()) } _, err = clientset.CoreV1().Pods(podNamespace).Create(context.Background(), pod, metav1.CreateOptions{}) if err != nil { panic(err.Error()) } fmt.Printf("Pod %s in namespace %s restarted\n", podName, podNamespace) } }
This code snippet first checks if the program is running inside a Kubernetes cluster by looking for the presence of a service account token file. If the program is running inside a cluster, it uses the in-cluster kubeconfig to create a Kubernetes clientset. Otherwise, it uses the kubeconfig file in the default location to create the clientset.
The code snippet then gets the pod with the given name and namespace using the clientset, and restarts the pod by deleting and creating a new pod with the same spec, similar to the previous code snippet.
Note that when using a service account, you do not need to provide the username and password for authentication, as the service account token and CA certificate are used instead. However, you need to make sure that the service account has the appropriate RBAC permissions to perform the necessary operations on the Kubernetes resources.
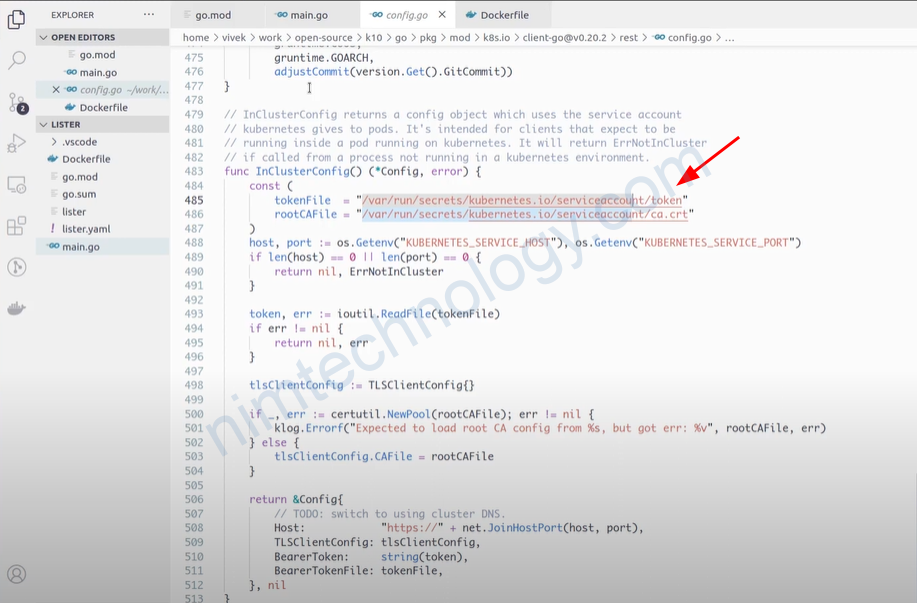
https://laury.dev/snippets/read-kubernetes-metrics-server-data-using-golang/