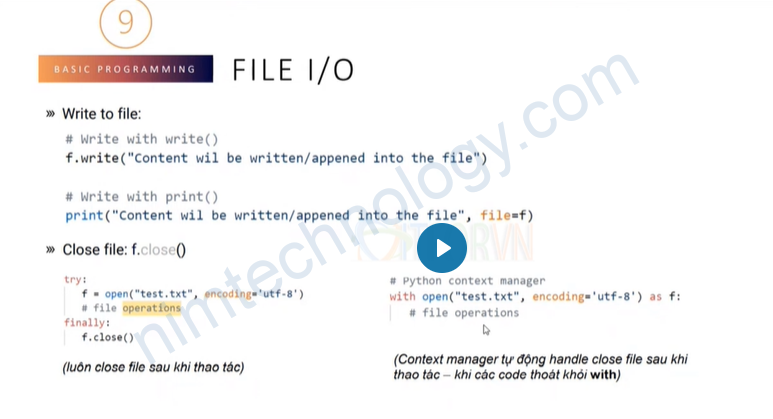
### Class class Human: """ This is Human prototype Created by GOD """ def __init__(self, name, color): self.name, self.color = name, color def greet(self): print("Hello, I'm Human, created by GOD") print(f"\tMy Name is: {self.name}") print(f"\tMy Color is: {self.color}") john = Human("Jonny Deep", "White") john.greet()
Đây là output
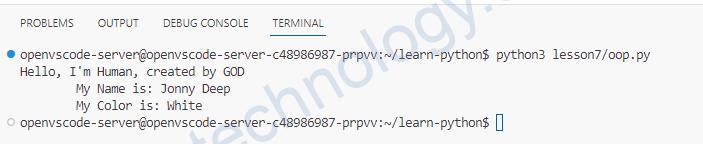
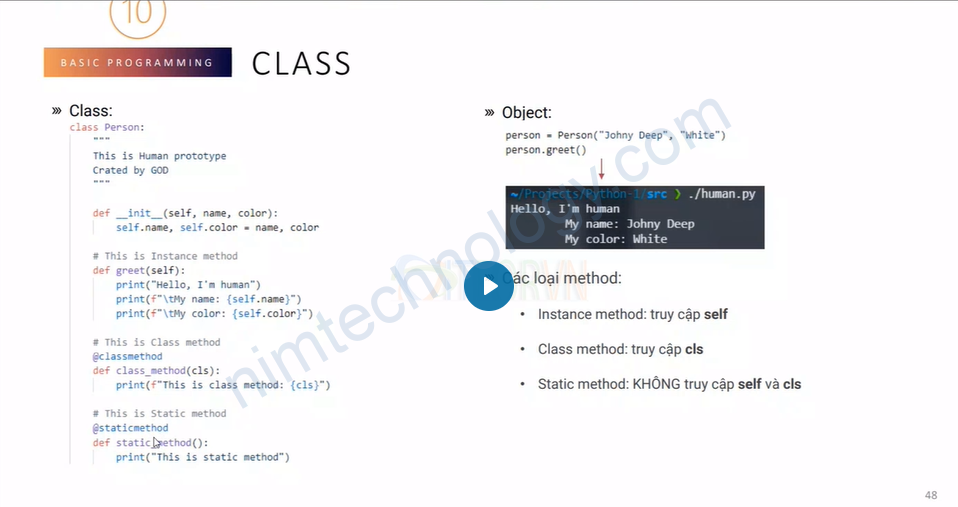
### Class class Human: """ This is Human prototype Created by GOD """ creator = "God" #class variable def __init__(self, name, color): self.name, self.color = name, color #Instance variable def greet(self): print(f"\tMy Name is: {self.name}") print(f"\tMy Color is: {self.color}") @classmethod def change_creator(cls, name) Human.creator = name john = Human("Jonny Deep", "White") print(f"{john.name} created by: {john.creator}") john.greet() nim = Human("Nim", "Red") print(f"{nim.name} created by: {nim.creator}") Human.creator = "Indra" lee = Human("Lee", "Black") print(f"{lee.name} created by: {lee.creator}")
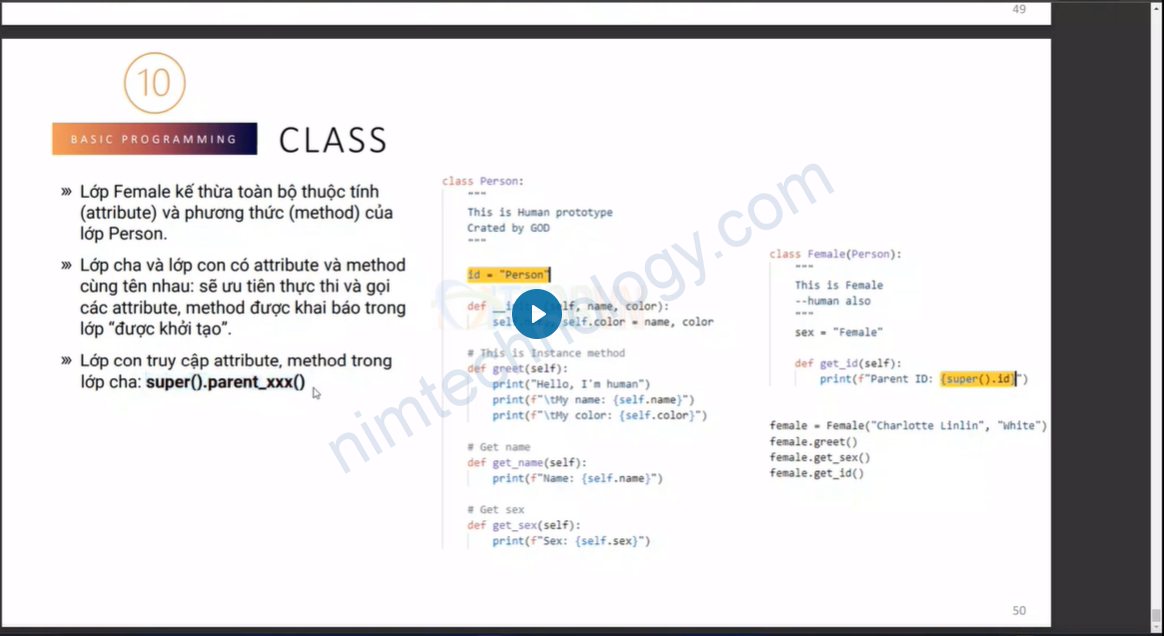
import random ### Class class Emloyee: company_name = "Nimtechnology" #class variable ceo = "Win" def __init__(self, name, color, country): self.name, self.color = name, color #Instance variable self.country = country #instance method def greet(self): print(f"Hello, I'm emloyee at {Emloyee.company_name}") print(f"\tMy Name is: {self.name}") print(f"\tMy Color is: {self.color}") print(f"\tMy country is: {self.country}") @classmethod def change_creator(cls, name): Emloyee.company_name = name @staticmethod def age(number): age_random = random.randrange(50,60) return age_random + number class EmployeeMarketing(Emloyee): department = "Marketing" class EmployeeIT(Emloyee): department = "IT" play_football = True def greet(self): print(f"Hello, I'm emloyee at {Emloyee.company_name} company") print(f"\tMy Name is: {self.name}") print(f"\tMy Color is: {self.color}") print(f"\tMy country is: {self.country}") print(f"\tPlay Football is: {EmployeeIT.play_football}") print(f"\tCEO is: {super().ceo}") john = EmployeeIT("Nim", "Red", "VietNam") john.greet()

Và output như trên