Basic level
Bình thường khi bạn sự dụng helm command để có thể install Keda hoặc là Argocd lên k8s bằng helm chart.
Giờ nếu bạn cần code 1 tool bằng golang để làm luôn chuyển đó thì như thể nào nhỉ?
Bạn có thể thao khảo các lệnh sau:
https://github.com/helm/helm-www/tree/main/sdkexamples
Chúng ta sẽ cúng tìm hiểu chi tiết hơn:
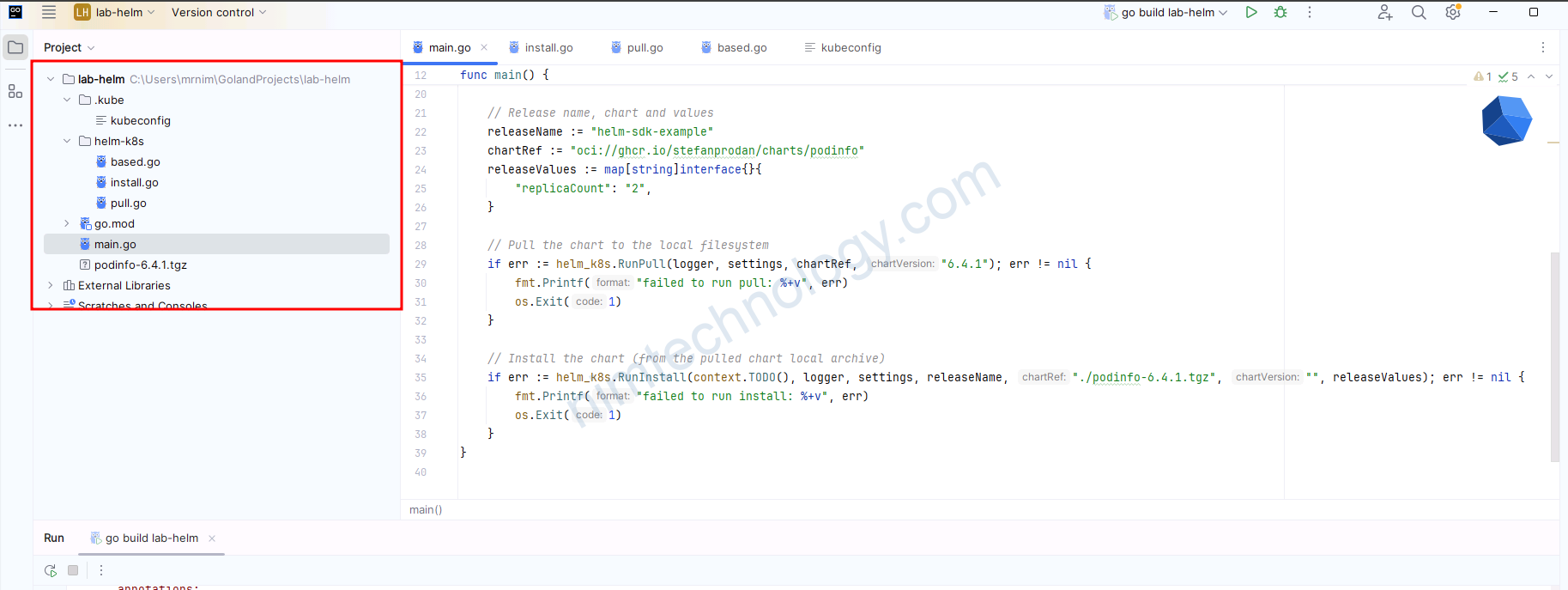
Trên đây là cấu trúc thư mục
đâu tiên là file main.go
package main import ( "context" "fmt" "helm.sh/helm/v3/pkg/cli" helm_k8s "lab-helm/helm-k8s" "log" "os" ) func main() { logger := log.Default() // For convenience, initialize SDK setting via CLI mechanism // Create a new CLI settings instance and override the KubeConfig path. settings := cli.New() settings.KubeConfig = ".kube/kubeconfig" // Release name, chart and values releaseName := "helm-sdk-example" chartRef := "oci://ghcr.io/stefanprodan/charts/podinfo" releaseValues := map[string]interface{}{ "replicaCount": "2", } // Pull the chart to the local filesystem if err := helm_k8s.RunPull(logger, settings, chartRef, "6.4.1"); err != nil { fmt.Printf("failed to run pull: %+v", err) os.Exit(1) } // Install the chart (from the pulled chart local archive) if err := helm_k8s.RunInstall(context.TODO(), logger, settings, releaseName, "./podinfo-6.4.1.tgz", "", releaseValues); err != nil { fmt.Printf("failed to run install: %+v", err) os.Exit(1) } }
Mặc định thì helm.sh/helm/v3/pkg/cli sẽ authen với k8s thông qua cách cánh sau:
1. Kubeconfig File:What it is: A configuration file that contains details about clusters, users, and contexts.
– Example: Located at ~/.kube/config by default.
– Usage: Helm reads this file to get the credentials needed to access a Kubernetes cluster.
2. Environment Variables:
– What they do: Override default settings or specify the location of the kubeconfig file.
– Example: Set KUBECONFIG=/path/to/your/config to use a specific kubeconfig file.
Nhưng nếu bạn muốn chủ động khai báo kubeconfig cho helm cli Library luôn thì bạn làm như sau:
// For convenience, initialize SDK setting via CLI mechanism // Create a new CLI settings instance and override the KubeConfig path. settings := cli.New() settings.KubeConfig = ".kube/kubeconfig"
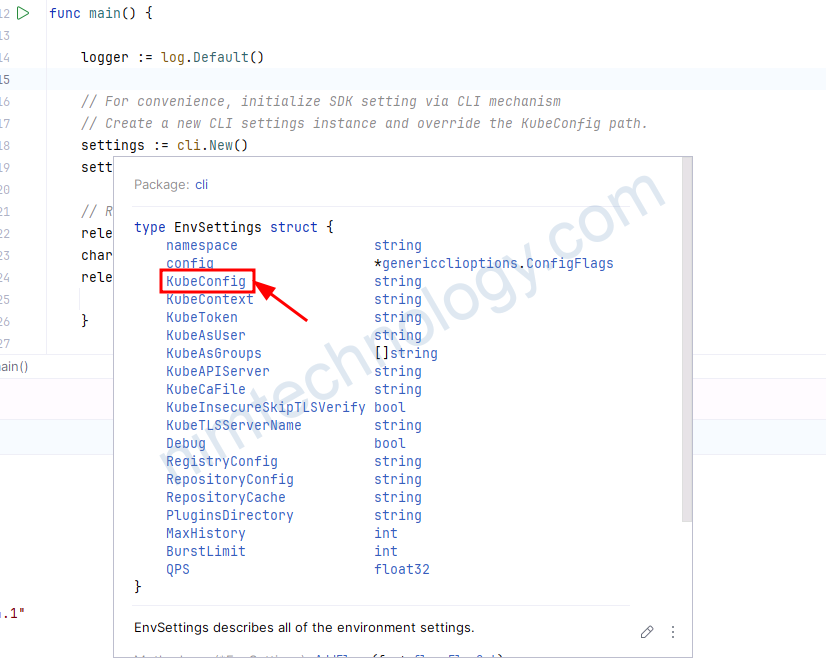
Bạn thấy trong EnvSettings có một parameter là Kubeconfig.
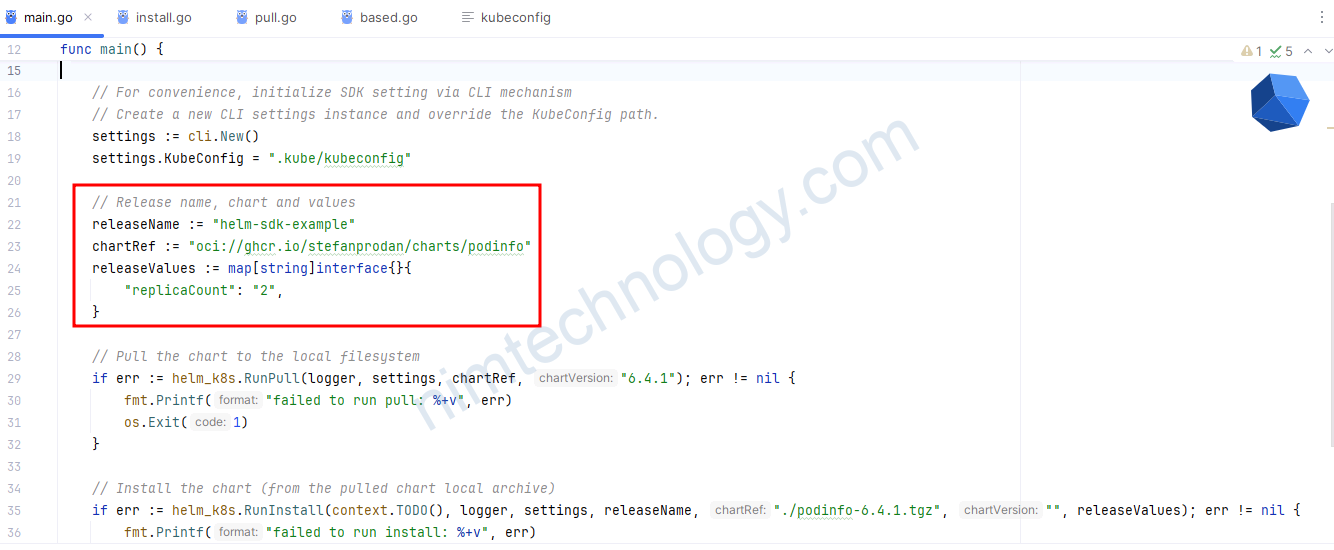
Bạn sẽ thấy các thông tin cơ bản như
Chart Name là gì?
Chart URL?
Helm value?
Khá là quên thuộc
Tiếp theo là mình có viết riêng 1 function để làm việc Pull Helm parckage.
package meta_structure import ( "mdaas-cli/logger" "helm.sh/helm/v3/pkg/action" "helm.sh/helm/v3/pkg/cli" ) func RunPull(settings *cli.EnvSettings, chartRef, chartVersion string) error { actionConfig, err := initActionConfig(settings) if err != nil { logger.Errorf("failed to init action config: %w", err) return err } registryClient, err := newRegistryClient(settings, false) if err != nil { logger.Errorf("failed to created registry client: %w", err) return err } actionConfig.RegistryClient = registryClient pullClient := action.NewPullWithOpts( action.WithConfig(actionConfig)) // client.RepoURL = "" pullClient.DestDir = "./mdaas_meta/" pullClient.Settings = settings pullClient.Version = chartVersion result, err := pullClient.Run(chartRef) if err != nil { logger.Errorf("failed to pull chart: %w", err) return err } logger.Debugf("Pull Helm chart Result: %+v", result) return nil }
Global Variable
var helmDriver string = os.Getenv("HELM_DRIVER")
helmDriver: This variable stores the value of the HELM_DRIVER environment variable. It determines the storage backend for Helm (e.g., configmaps, secrets).
Function: initActionConfig
Purpose: Initializes an action.Configuration object, which is used to configure Helm actions.
func initActionConfig(settings *cli.EnvSettings, logger *log.Logger) (*action.Configuration, error) { return initActionConfigList(settings, logger, false) }
Mục đích: Khởi tạo cấu hình hành động (action.Configuration) cho Helm.
Tham số:
– settings: Cài đặt môi trường cho CLI của Helm.
– logger: Đối tượng ghi nhật ký để ghi thông tin.
Hoạt động: Gọi hàm initActionConfigList với tham số allNamespaces là false, nghĩa là chỉ khởi tạo cho một namespace cụ thể.
Function: initActionConfigList
func initActionConfigList(settings *cli.EnvSettings, logger *log.Logger, allNamespaces bool) (*action.Configuration, error) { actionConfig := new(action.Configuration) namespace := func() string { if allNamespaces { return "" } return settings.Namespace() }() if err := actionConfig.Init( settings.RESTClientGetter(), namespace, helmDriver, logger.Printf); err != nil { return nil, err } return actionConfig, nil }
Mục đích: Khởi tạo cấu hình hành động cho Helm, có thể cho tất cả các namespace hoặc chỉ một namespace cụ thể.
Tham số:
– settings: Cài đặt môi trường cho CLI của Helm.
– logger: Đối tượng ghi nhật ký.all
– Namespaces: Boolean xác định có khởi tạo cho tất cả các namespace hay không.
Hoạt động:
– Tạo một đối tượng action.Configuration.
– Xác định namespace dựa trên giá trị của allNamespaces.
– Khởi tạo cấu hình với các thông số từ settings và logger.
– Trả về cấu hình đã khởi tạo hoặc lỗi nếu có.
Trong Funtion registryClient, err := newRegistryClient(settings, false)
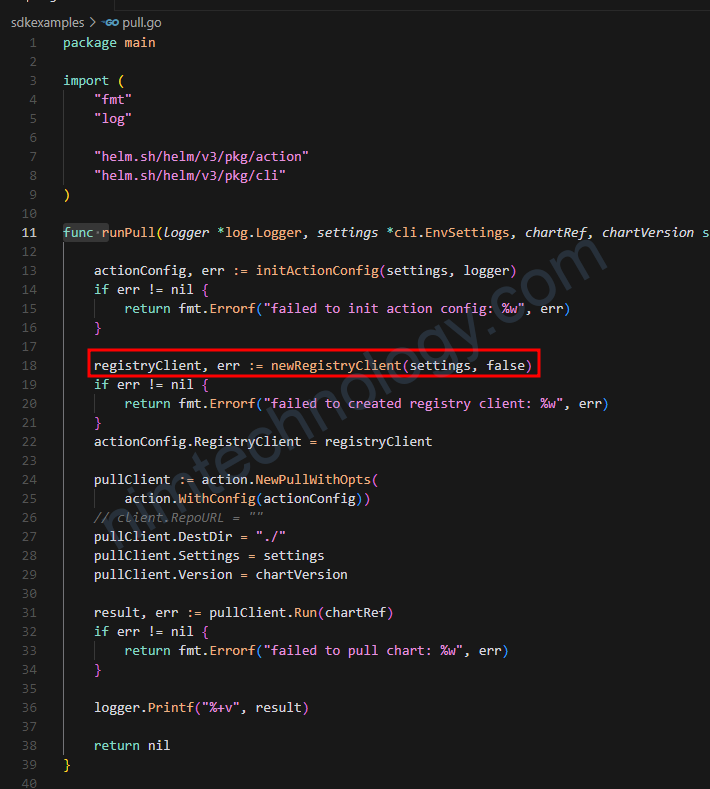
Chúng ta cùng tìm hiểu logic của funtion:
func newRegistryClient(settings *cli.EnvSettings, plainHTTP bool) (*registry.Client, error) { opts := []registry.ClientOption{ registry.ClientOptDebug(settings.Debug), registry.ClientOptEnableCache(true), registry.ClientOptWriter(os.Stderr), registry.ClientOptCredentialsFile(settings.RegistryConfig), } if plainHTTP { opts = append(opts, registry.ClientOptPlainHTTP()) } registryClient, err := registry.NewClient(opts...) if err != nil { return nil, err } return registryClient, nil }
Mục đích: Tạo một client để tương tác với registry của Helm.
Tham số:
– settings: Cài đặt môi trường cho CLI của Helm.
– plainHTTP: Boolean xác định có sử dụng HTTP không mã hóa hay không.
Hoạt động:
– Tạo danh sách các tùy chọn cho client, bao gồm chế độ gỡ lỗi, bộ nhớ đệm, và tệp thông tin xác thực.
– Nếu plainHTTP là true, thêm tùy chọn sử dụng HTTP không mã hóa.
– Tạo và trả về một đối tượng registry.Client hoặc lỗi nếu có.
Tiếp theo chúng ta chuyển qua RunIstall
Chúng ta cần tìm hiểu newRegistryClientTLS
func newRegistryClientTLS(settings *cli.EnvSettings, logger *log.Logger, certFile, keyFile, caFile string, insecureSkipTLSverify, plainHTTP bool) (*registry.Client, error) { if certFile != "" && keyFile != "" || caFile != "" || insecureSkipTLSverify { registryClient, err := registry.NewRegistryClientWithTLS( logger.Writer(), certFile, keyFile, caFile, insecureSkipTLSverify, settings.RegistryConfig, settings.Debug) if err != nil { return nil, err } return registryClient, nil } registryClient, err := newRegistryClient(settings, plainHTTP) if err != nil { return nil, err } return registryClient, nil }
Mục đích: Tạo một client để tương tác với registry của Helm, hỗ trợ TLS.
Tham số:
– settings: Cài đặt môi trường cho CLI của Helm.
– logger: Đối tượng ghi nhật ký.
– certFile, keyFile, caFile: Các tệp chứng chỉ và khóa cho TLS.
– insecureSkipTLSverify: Boolean xác định có bỏ qua xác minh TLS hay không.
– plainHTTP: Boolean xác định có sử dụng HTTP không mã hóa hay không.
Hoạt động:
– Nếu có thông tin TLS, tạo client với hỗ trợ TLS.
– Nếu không, tạo client thông thường bằng cách gọi newRegistryClient.
Quay trở lại với runInstall Funtion
Mục đích: Hàm này thực hiện việc cài đặt một biểu đồ Helm (Helm chart) với các thông số được cung cấp.
Tham số:
- ctx: Context để quản lý vòng đời của các yêu cầu.
- logger: Đối tượng logger để ghi lại các thông điệp log.
- settings: Cài đặt môi trường CLI của Helm.
- releaseName: Tên của bản phát hành (release) sẽ được tạo.
- chartRef: Tham chiếu đến biểu đồ Helm cần cài đặt.
- chartVersion: Phiên bản của biểu đồ Helm.
- releaseValues: Các giá trị cấu hình cho bản phát hành.
Quá trình:
- Khởi tạo cấu hình hành động (actionConfig) và kiểm tra lỗi.
- Tạo một đối tượng cài đặt (installClient) từ cấu hình hành động.
- Thiết lập các thuộc tính cho installClient như tên bản phát hành, không gian tên, và phiên bản biểu đồ.
- Tạo một client registry với các thông tin TLS và thiết lập cho installClient.
- Xác định đường dẫn của biểu đồ Helm và tải biểu đồ từ đường dẫn đó.Kiểm tra và cập nhật các phụ thuộc của biểu đồ nếu cần thiết.
- Thực hiện cài đặt biểu đồ với các giá trị cấu hình và ghi lại thông tin bản phát hành.
Investigate Helm’s Golang interaction with both standard Helm and OCI Helm.
Standard Helm
Với kiểu helm chart mặc định thì chúng ta có funtion sau:
// InstallOrUpgradeChartNormal installs or upgrades a normal (HTTP-based) Helm chart. func InstallOrUpgradeChartNormal(logger *log.Logger, chartRepoURL, chartName, chartVersion, namespace, releaseName string, releaseValues map[string]interface{}) (*release.Release, error) { settings := cli.New() settings.SetNamespace(namespace) // Optionally specify the kubeconfig location if needed. settings.KubeConfig = ".kube/kubeconfig" actionConfig := new(action.Configuration) if err := actionConfig.Init(settings.RESTClientGetter(), namespace, os.Getenv("HELM_DRIVER"), logger.Printf); err != nil { return nil, fmt.Errorf("failed to initialize helm action configuration: %w", err) } // Ensure the URL is not for an OCI chart. if strings.HasPrefix(chartRepoURL, "oci://") { return nil, fmt.Errorf("chartRepoURL is OCI; please use InstallOrUpgradeChartOCI instead") } // Create a repo entry and download the repository index. repoEntry := &repo.Entry{ Name: chartName, URL: chartRepoURL, } chartRepo, err := repo.NewChartRepository(repoEntry, getter.All(settings)) if err != nil { return nil, fmt.Errorf("failed to create chart repository client: %w", err) } if _, err = chartRepo.DownloadIndexFile(); err != nil { return nil, fmt.Errorf("failed to download chart repository index: %w", err) } // Check if the release exists. histClient := action.NewHistory(actionConfig) _, err = histClient.Run(releaseName) isInstall := err == driver.ErrReleaseNotFound if err != nil && !isInstall { return nil, fmt.Errorf("error checking release history: %w", err) } // Locate and load the chart. chartPathOptions := action.ChartPathOptions{ RepoURL: chartRepoURL, Version: chartVersion, } chartPath, err := chartPathOptions.LocateChart(chartName, settings) if err != nil { return nil, fmt.Errorf("failed to locate chart: %w", err) } chartRequested, err := loader.Load(chartPath) if err != nil { return nil, fmt.Errorf("failed to load chart: %w", err) } // Install or upgrade the release. if isInstall { logger.Printf("Release %s not found, proceeding with installation...\n", releaseName) installClient := action.NewInstall(actionConfig) installClient.ReleaseName = releaseName installClient.Namespace = namespace installClient.CreateNamespace = true installClient.Version = chartVersion return installClient.Run(chartRequested, releaseValues) } logger.Printf("Release %s found, proceeding with upgrade...\n", releaseName) upgradeClient := action.NewUpgrade(actionConfig) upgradeClient.Namespace = namespace upgradeClient.Version = chartVersion return upgradeClient.Run(releaseName, chartRequested, releaseValues) }
OCI Helm chart
func InstallOrUpgradeChartOCI(logger *log.Logger, chartRepoURL, chartName, chartVersion, namespace, releaseName string, releaseValues map[string]interface{}) (*release.Release, error) { settings := cli.New() settings.SetNamespace(namespace) settings.KubeConfig = ".kube/kubeconfig" actionConfig := new(action.Configuration) if err := actionConfig.Init(settings.RESTClientGetter(), namespace, os.Getenv("HELM_DRIVER"), logger.Printf); err != nil { return nil, fmt.Errorf("failed to initialize helm action configuration: %w", err) } // Construct the full OCI chart reference. chartRef := fmt.Sprintf("%s/%s", chartRepoURL, chartName) // Check if the release exists. histClient := action.NewHistory(actionConfig) _, err := histClient.Run(releaseName) isInstall := err == driver.ErrReleaseNotFound if err != nil && !isInstall { return nil, fmt.Errorf("error checking release history: %w", err) } // Always create the registry client. registryClient, err := registry.NewClient( registry.ClientOptDebug(true), registry.ClientOptEnableCache(true), ) if err != nil { return nil, fmt.Errorf("failed to create registry client: %w", err) } if isInstall { logger.Printf("Release %s not found, proceeding with installation...\n", releaseName) installClient := action.NewInstall(actionConfig) installClient.ReleaseName = releaseName installClient.Namespace = namespace installClient.CreateNamespace = true installClient.Version = chartVersion // Set the registry client. installClient.SetRegistryClient(registryClient) chartPath, err := installClient.LocateChart(chartRef, settings) if err != nil { return nil, fmt.Errorf("failed to locate chart: %w", err) } chartRequested, err := loader.Load(chartPath) if err != nil { return nil, fmt.Errorf("failed to load chart: %w", err) } return installClient.Run(chartRequested, releaseValues) } logger.Printf("Release %s found, proceeding with upgrade...\n", releaseName) upgradeClient := action.NewUpgrade(actionConfig) upgradeClient.Namespace = namespace upgradeClient.Version = chartVersion upgradeClient.SetRegistryClient(registryClient) chartPath, err := upgradeClient.LocateChart(chartRef, settings) if err != nil { return nil, fmt.Errorf("failed to locate chart: %w", err) } chartRequested, err := loader.Load(chartPath) if err != nil { return nil, fmt.Errorf("failed to load chart: %w", err) } return upgradeClient.Run(releaseName, chartRequested, releaseValues) }
Installing Many Helm Charts via Golang.
You want to create a configuration file to store multiple ChartInstallConfig objects, then loop through them to install each chart. This is a good approach for managing multiple chart installations.
Let’s design this solution:
1) Define a structure for the configuration file.
2) Create functions to load the configuration file
3) Implement a loop to install each chart
For the configuration file, we can use YAML or JSON – YAML is very common in Kubernetes contexts, so I’ll go with that. The config structure would essentially be an array of ChartInstallConfig objects.
1. Create a Configuration Structure
First, let’s define the structure for our configuration file:
package main import ( "fmt" "io/ioutil" "os" "path/filepath" "gopkg.in/yaml.v2" "helm.sh/helm/v3/pkg/release" ) // ChartInstallConfig encapsulates the parameters needed for installing // or upgrading a Helm chart type ChartInstallConfig struct { KubeConfigPath string `yaml:"kubeConfigPath"` ChartRepoURL string `yaml:"chartRepoURL"` ChartName string `yaml:"chartName"` ReleaseName string `yaml:"releaseName"` ChartVersion string `yaml:"chartVersion"` Namespace string `yaml:"namespace"` ReleaseValues map[string]interface{} `yaml:"releaseValues,omitempty"` } // ChartsConfig represents the overall configuration containing multiple chart configs type ChartsConfig struct { Charts []ChartInstallConfig `yaml:"charts"` }
2. Function to Load the Configuration
// LoadChartsConfig loads chart configurations from a YAML file func LoadChartsConfig(configPath string) (*ChartsConfig, error) { // Ensure the file exists _, err := os.Stat(configPath) if os.IsNotExist(err) { return nil, fmt.Errorf("configuration file %s does not exist", configPath) } // Read the file data, err := ioutil.ReadFile(configPath) if err != nil { return nil, fmt.Errorf("failed to read configuration file: %w", err) } // Parse YAML var config ChartsConfig if err := yaml.Unmarshal(data, &config); err != nil { return nil, fmt.Errorf("failed to parse configuration: %w", err) } // Convert the releaseValues to the correct type for each chart for i := range config.Charts { if config.Charts[i].ReleaseValues != nil { config.Charts[i].ReleaseValues = convertToStringMap(config.Charts[i].ReleaseValues) } } // Validate the configuration for i, chart := range config.Charts { if chart.ChartName == "" { return nil, fmt.Errorf("chart at index %d is missing required field 'chartName'", i) } if chart.ChartRepoURL == "" { return nil, fmt.Errorf("chart at index %d is missing required field 'chartRepoURL'", i) } if chart.ReleaseName == "" { return nil, fmt.Errorf("chart at index %d is missing required field 'releaseName'", i) } // Set default namespace if not provided if chart.Namespace == "" { config.Charts[i].Namespace = "default" } } return &config, nil } // convertToStringMap recursively converts map[interface{}]interface{} to map[string]interface{} // This is needed because YAML unmarshaling creates map[interface{}]interface{}, // but Helm expects map[string]interface{} func convertToStringMap(m map[string]interface{}) map[string]interface{} { result := make(map[string]interface{}) for k, v := range m { switch value := v.(type) { case map[interface{}]interface{}: // Convert keys to strings strMap := make(map[string]interface{}) for k2, v2 := range value { strMap[fmt.Sprintf("%v", k2)] = v2 } // Recursively convert nested maps result[k] = convertToStringMap(strMap) case map[string]interface{}: // Recursively convert nested maps result[k] = convertToStringMap(value) case []interface{}: // Convert slice elements that are maps newSlice := make([]interface{}, len(value)) for i, item := range value { if mapItem, ok := item.(map[interface{}]interface{}); ok { strMap := make(map[string]interface{}) for k2, v2 := range mapItem { strMap[fmt.Sprintf("%v", k2)] = v2 } newSlice[i] = convertToStringMap(strMap) } else if mapItem, ok := item.(map[string]interface{}); ok { newSlice[i] = convertToStringMap(mapItem) } else { newSlice[i] = item } } result[k] = newSlice default: result[k] = v } } return result }
Understanding the convertToStringMap Function
The convertToStringMap function solves a very specific problem that occurs when working with YAML in Go. Let me explain it in a way that’s easier to understand:
The Problem
When Go reads YAML files, it creates maps where keys can be of any type: (map[interface{}]interface{})
.
However, Helm and JSON encoding only accept maps where keys are strings (map[string]interface{})
.
This mismatch causes the error: unsupported type: map[interface{}]interface{}
What This Function Does
The function takes a map and ensures all keys throughout the entire structure (including nested maps) are strings:
func convertToStringMap(m map[string]interface{}) map[string]interface{} { result := make(map[string]interface{}) for k, v := range m { // Process each value based on its type switch value := v.(type) { // Case 1: If we find a map with interface{} keys case map[interface{}]interface{}: // Create a new map with string keys strMap := make(map[string]interface{}) // Convert each key to a string for k2, v2 := range value { strMap[fmt.Sprintf("%v", k2)] = v2 } // Process this map recursively to handle deeper nesting result[k] = convertToStringMap(strMap) // Case 2: If we find a regular map with string keys that might contain nested maps case map[string]interface{}: // Process this map recursively result[k] = convertToStringMap(value) // Case 3: If we find an array/slice that might contain maps case []interface{}: // Create a new slice to hold converted items newSlice := make([]interface{}, len(value)) // Process each item in the slice for i, item := range value { if mapItem, ok := item.(map[interface{}]interface{}); ok { // Convert map with interface{} keys to map with string keys strMap := make(map[string]interface{}) for k2, v2 := range mapItem { strMap[fmt.Sprintf("%v", k2)] = v2 } newSlice[i] = convertToStringMap(strMap) } else if mapItem, ok := item.(map[string]interface{}); ok { // Process regular map recursively newSlice[i] = convertToStringMap(mapItem) } else { // Keep non-map values unchanged newSlice[i] = item } } result[k] = newSlice // Case 4: For all other types (strings, numbers, booleans, etc.) default: // Keep them as they are result[k] = v } } return result }
Example to Illustrate
Let’s see what happens with your example YAML:
metrics-server: image: repository: registry.k8s.io/metrics-server/metrics-server metrics: enabled: true
When parsed with Go’s YAML library, it becomes something like:
map[string]interface{}{ "metrics-server": map[interface{}]interface{}{ "image": map[interface{}]interface{}{ "repository": "registry.k8s.io/metrics-server/metrics-server", }, "metrics": map[interface{}]interface{}{ "enabled": true, }, }, }
After running through convertToStringMap, it becomes:
map[string]interface{}{ "metrics-server": map[string]interface{}{ "image": map[string]interface{}{ "repository": "registry.k8s.io/metrics-server/metrics-server", }, "metrics": map[string]interface{}{ "enabled": true, }, }, }
Now all map keys are strings, which makes Helm and JSON processing happy!
In Simple Terms
Think of it like translating a document where some words are in a language your application doesn’t understand. The function walks through the entire document, finds those words, and translates them to a language your application does understand (string keys), while keeping the meaning (values) intact.
3. Main Function to Install All Charts
// InstallAllCharts installs or upgrades all charts from the config func InstallAllCharts(configPath string) error { // Load the configuration config, err := LoadChartsConfig(configPath) if err != nil { return fmt.Errorf("failed to load charts configuration: %w", err) } // Track any installation failures var failures []string // Install each chart for i, chartConfig := range config.Charts { logger.Infof("Installing chart %d/%d: %s", i+1, len(config.Charts), chartConfig.ChartName) // Use the existing InstallOrUpgradeChartNormal function _, err := InstallOrUpgradeChartNormal( chartConfig.KubeConfigPath, chartConfig.ChartRepoURL, chartConfig.ChartName, chartConfig.ReleaseName, chartConfig.ChartVersion, chartConfig.Namespace, chartConfig.ReleaseValues, ) if err != nil { failures = append(failures, fmt.Sprintf("%s: %v", chartConfig.ChartName, err)) logger.Errorf("Failed to install chart %s: %v", chartConfig.ChartName, err) // Continue with the next chart even if this one failed continue } logger.Infof("Successfully installed/upgraded chart: %s", chartConfig.ChartName) } // Report any failures if len(failures) > 0 { return fmt.Errorf("failed to install %d charts: %v", len(failures), failures) } return nil }
Example Configuration File (charts-config.yaml)
charts: - chartName: keda chartRepoURL: https://kedacore.github.io/charts releaseName: keda chartVersion: 2.10.1 namespace: keda # releaseValues field is omitted (will be nil) - chartName: prometheus chartRepoURL: https://prometheus-community.github.io/helm-charts releaseName: prometheus chartVersion: 15.10.1 namespace: monitoring releaseValues: server: persistentVolume: size: 20Gi alertmanager: enabled: true - chartName: nginx-ingress chartRepoURL: https://kubernetes.github.io/ingress-nginx releaseName: nginx-ingress chartVersion: 4.3.0 namespace: ingress-nginx releaseValues: controller: replicaCount: 2 service: type: LoadBalancer
Command-Line Interface to Run Installations
package main import ( "flag" "fmt" "os" ) func main() { // Parse command-line arguments configPath := flag.String("config", "", "Path to the charts configuration file") flag.Parse() if *configPath == "" { fmt.Println("Error: Missing required -config flag") fmt.Println("Usage: program -config /path/to/charts-config.yaml") os.Exit(1) } // Install all charts from the configuration if err := InstallAllCharts(*configPath); err != nil { fmt.Printf("Error installing charts: %v\n", err) os.Exit(1) } fmt.Println("All charts have been successfully processed") }